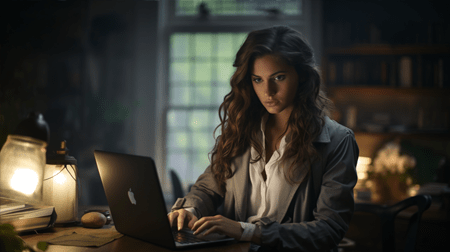
Understanding Variables in JavaScript: A Comprehensive Guide for Beginners
July 22, 2023
Active Directory Penetration Testing: A Pro’s Guide
July 22, 2023A Comprehensive Tutorial on Data Classification in JavaScript
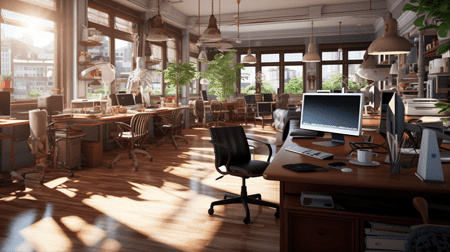
Data classification is a key concept in the world of machine learning, and it refers to the process of categorizing data into various predefined classes. It is especially useful when we need to make predictions or decisions based on the provided data. In this tutorial, we’ll explore how to implement data classification in JavaScript, making use of libraries such as TensorFlow.js. Despite being a language commonly associated with front-end web development, JavaScript’s capabilities extend to data science tasks too.
Setting Up Your Environment
First, we need to set up our environment. You’ll need Node.js installed on your machine, which you can download from here. Once installed, verify the installation by typing the following commands in your terminal:
node -v
npm -v
Next, we install TensorFlow.js using npm:
npm install @tensorflow/tfjs
Implementing Classification with TensorFlow.js
Once we have TensorFlow.js installed, we can proceed with the data classification.
Firstly, we need to import the TensorFlow.js library:
const tf = require('@tensorflow/tfjs');
Let’s assume we have a simple dataset that we want to classify. For simplicity, this tutorial will use a binary classification problem – classifying data into either class 0 or class 1.
const data = [
{features: [1, 2], label: 0},
{features: [2, 3], label: 0},
{features: [3, 4], label: 1},
{features: [4, 5], label: 1},
];
Here, ‘features’ are the data attributes, and ‘label’ is the class to which the data belongs.
To make this data usable for TensorFlow.js, we need to convert it to Tensors:
const convertedData = data.map(item => {
return {
xs: tf.tensor1d(item.features),
ys: tf.tensor1d([item.label])
}
});
Next, we create a sequential model with TensorFlow.js:
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [2]}));
Then, we compile the model:
model.compile({loss: 'meanSquaredError', optimizer: 'sgd'});
Finally, we can train the model:
(async function() {
for (let i = 0; i < 10; i++) {
const response = await model.fitDataset(convertedData, {
epochs: 10,
});
console.log(response.history.loss[0]);
}
})();
With the code above, we train our model for ten epochs. An epoch is an entire cycle through the full training dataset.
Once the model is trained, we can use it to make predictions:
const output = model.predict(tf.tensor2d([5, 6], [1, 2]));
output.print();
Conclusion
Data classification plays a pivotal role in machine learning, enabling us to make informed decisions and predictions. In this tutorial, we saw how to perform data classification using JavaScript and TensorFlow.js. Although JavaScript might not be the first language that comes to mind for data science tasks, with the right tools and libraries, it is more than capable of performing these tasks effectively.