
The Pro Pentester’s Toolkit: Essential Hacking Tools for 2023
July 23, 2023
Understanding XSS (Cross-Site Scripting)
July 27, 2023Mastering the If-Else Operator in JavaScript: A Comprehensive Tutorial for Developers
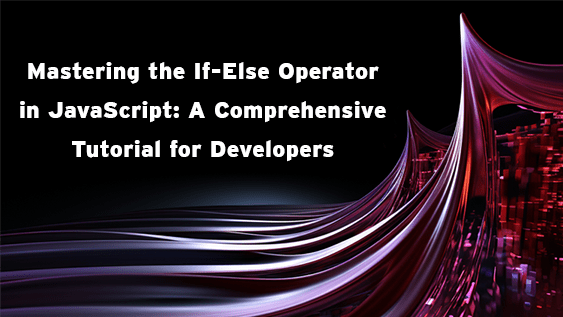
Mastering the If-Else Operator in JavaScript A Comprehensive Tutorial for Developers
JavaScript has established itself as a powerful language for web development. One of its essential elements is the control flow statements, among which the “If-Else” operator stands out due to its straightforwardness and extensive utility. This article will serve as a tutorial to master the If-Else operator in JavaScript.
Introduction
Before diving in, it’s important to understand that control flow is the order in which individual statements, instructions, or function calls are executed within a script. In JavaScript, control structures such as “If-Else” statements allow developers to create branches in their code, which can execute different blocks of code based on certain conditions.
Understanding the If-Else Operator
The If-Else statement is a common conditional statement in many programming languages, including JavaScript. It evaluates an expression for a Boolean value (either true or false) and then executes a certain block of code based on that outcome.
The syntax of an If-Else statement in JavaScript is as follows:
if (condition) {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
The condition within the parentheses after the if
keyword is what the JavaScript engine evaluates. If the condition is true, the code block within the braces after the if
statement is executed. If the condition is false, the code block within the braces after the else
keyword is executed.
Example of If-Else Operator
Let’s look at a simple example:
let number = 15;
if (number > 10) {
console.log('The number is greater than 10.');
} else {
console.log('The number is not greater than 10.');
}
In the example above, the condition checks whether the number is greater than 10. Since 15 is indeed greater than 10, ‘The number is greater than 10.’ is displayed in the console.
The “Else If” Condition
Sometimes, you might want to check multiple conditions. In such cases, you can use the else if
condition. This condition can be used to include additional checks after the if
statement.
The syntax for an else if
statement is:
if (condition1) {
// Code to execute if condition1 is true
} else if (condition2) {
// Code to execute if condition1 is false and condition2 is true
} else {
// Code to execute if both condition1 and condition2 are false
}
Example of Else If Condition
Here’s a simple example to understand the else if
condition:
let number = 15;
if (number > 20) {
console.log('The number is greater than 20.');
} else if (number > 10) {
console.log('The number is greater than 10.');
} else {
console.log('The number is not greater than 10.');
}
In the example above, first, it checks if the number is greater than 20. If it’s false, then it checks the next condition – if the number is greater than 10. In this case, as the number 15 is not greater than 20 but is greater than 10, the output would be ‘The number is greater than 10.’
Conclusion
Mastering the use of If-Else statements can significantly improve your ability to write efficient and effective code in JavaScript. Practice writing If-Else statements, and before you know it, you will find yourself writing more complex JavaScript code with ease.